

Let us define our class template for the Queue implementation.įront and rear instance variable are the linked list node that holds the data and a reference to the next node. In a linked queue, each node of the queue consists of two parts i.e. once we remove the tail then there is no way to point to the element that was before a tail in a singly linked list unless we have an extra pointer. Removal of an element happens from the front of the Q and if the linked list tail is the front of a queue then how we going to remove the front element of a Q(the tail of linked list) as the linked list does not allow to remove the tail of it at a constant time O(1). The front of Q is the head and rear of the Q is tail due to the remove operation. The difference is that we will be using the Linked List data structure instead of Array. We will implement the same methods enqueue, dequeue, front, and display in this program. You can visit my previous article for custom implementation of linked list in Java. As we have implemented the Queue data structure using Arrays in the above program, we can also implement the Queue using Linked List. While using linked list for the queue implementation, EnQueue operation is implemented by inserting element at the end of the list and DeQueue operation is implemented by deleting an element from the beginning of the list. Every operation uses extra space and time to deal wih references.Every operation takes constant time O(1).Below are the advantages of using linked list for queue implementation:
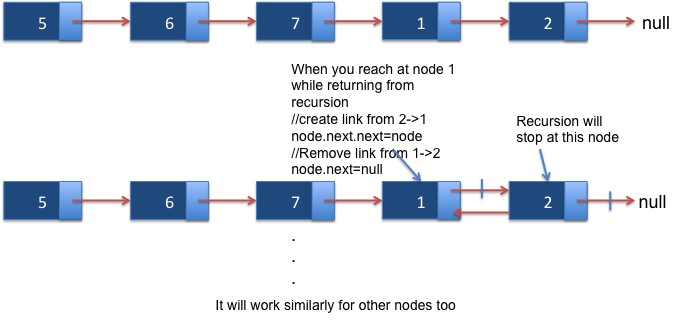
We will be using linked list for the implementation of our custom queue. IsEmpty(): Indicates whether no elements are stored in the queue or not. QueueSize(): Returns the number of elements stored in the queue. Queue OperationsĮnQueue(): Inserts an element at the end of the Queue.ĭeQueue(): Removes and returns the element at the front of the Queue.įront(): Returns the element at front without removing it. Hence, it is called First in First out(FIFO). The element which is inserted first is the first element that is deleted. What is QueueĪ queue is an ordered list in which insertions are done at one end(rear) and deletions are done at the other end(front). We will also check the time complexity of these different operations. In the course, we will perform different queue operations such as enQueue(), deQueue(), etc.
#Java queue linked list how to
Now since you know how to implement a queue using the LinkedList class, check out how to create a Queue using ArrayDeque.In this article, we will create a custom implementation of Queue data structure in Java. The methods offer(E element), element(), and remove() function similar to the methods add(E element), peek(), and poll() respectively but they don’t throw any exception if insertion is not possible, or return null if the queue is empty. It returns true if the queue is empty or false otherwise. enQueue (): This operation adds a new node after the rear and moves the rear to the next node. The front points to the first item of the queue and rear points to the last item. In other words, it removes and returns the first element of this list. Try It Approach: To solve the problem follow the below idea: we maintain two pointers, front, and rear. It retrieves and removes the head of this queue. It retrieves, but does not remove, the head of this queue (first element of this list). If no space is currently available, this method throws an 'IllegalStateException'. It returns true if the insertion is successful, or false if it is not. This method inserts an element at the end of the queue represented by this list. Let us look at the methods available to us.
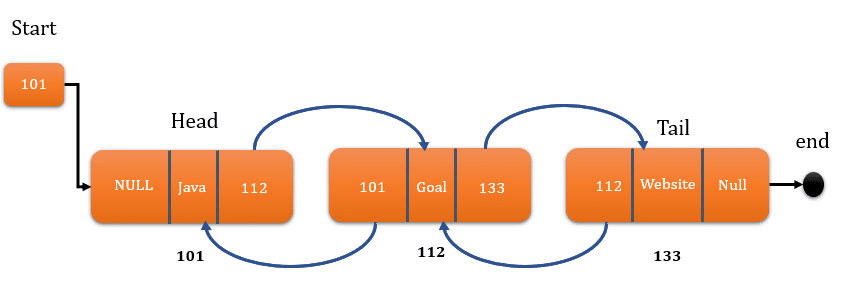

Example import public class Main Output Current element at the top of the queue: 2 Element removed from the queue: 2 Current elements in the queue: 5 6 The following example demonstrates how to implement a queue using the LinkedList class. But it is not recommended to insert nulls because null is used as a special return value by various methods to indicate that the deque is empty. LinkedList class permits all elements, including null. Some important methods provided by the LinkedList class for implementing a queue are: For an overview of the Java Collections Framework, check out my post Overview of the Java Collections Framework. Java provides the LinkedList class as part of the Java Collections Framework. In this post, we’ll see how to implement a queue using the LinkedList class. Queue is a linear data structure which orders elements in a First-In-First-Out (FIFO) manner, where the first element inserted is the first one to be removed.
